Spring button animation in iOS/Swift
In my app I have a big Play button. I wanted to show it with a nice bouncing effect to make it more noticeable and less boring.
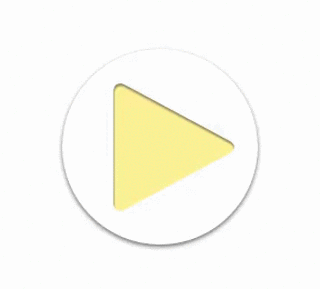
This kind of animation is easy to create with just a few lines of code. First, I made the button smaller by applying scale transformation:
button.transform = CGAffineTransform(scaleX: 0.1, y: 0.1)
Then I used spring style animation that resets the button to its initial state.
UIView.animate(withDuration: 2.0,
delay: 0,
usingSpringWithDamping: 0.2,
initialSpringVelocity: 6.0,
options: .allowUserInteraction,
animations: { [weak self] in
self?.button.transform = .identity
},
completion: nil)
Animation parameters
- First one is duration of the animation, which I set to 2 seconds.
usingSpringWithDamping
sets the wobbliness of the spring animation. The closer the value to zero the more shaky the animation.initialSpringVelocity
defines how fast the animation shoots out in the beginning.
It took me many minutes to play with those 3 parameters before I achieved the animation effect I liked.
Source files
- Demo app on GitHub
- Button image source created with Sketch app.
- Spring button animation tutorial for Android.
Posted by Evgenii